TikTok has surprised social media platforms with their billion plus active users worldwide as of 2023. Its unique format of short, engaging video posts has captured the attention of diverse audiences, from teenagers to adults, and even brands. The platform's algorithm is designed to surface content that is highly relevant to each user, making it an excellent venue for marketers and influencers to reach their target audiences effectively.
Understanding the nuances of audience engagement through comments is crucial for several reasons. Comments can reveal the emotional pulse of your audience, providing insights into their likes, dislikes, and overall sentiment towards your content posts. For brands, this can translate into valuable feedback on products or services, allowing them to make informed decisions on future marketing strategies. For influencers, it can guide content creation, helping them to stay relevant and connected with their followers.
This comprehensive guide delves into how to leverage a TikTok comment scraper, utilizing TikTok’s API, residential proxies and a suitable programming language, like Python, to extract data from TikTok and analyze comments efficiently, including video details, while ensuring that personally identifiable information (PII) is handled with care. User input is crucial in tailoring the scraper to meet specific needs.
Understanding the Importance of Scraping TikTok Comments
Comments on TikTok videos are rich data points that offer insights into user engagement, preferences, and emerging trends. Monitoring the comment count can help gauge the popularity and impact of your content. TikTok data scraping allows for a granular analysis of user feedback, which can enhance data-related efforts such as content strategy, market research, and customer engagement. tilizing all available resources effectively can maximize these benefits.
The Role of Comments in User Engagement
TikTok comments provide qualitative data that likes and shares simply cannot. They reveal the emotional responses of TikTok users, highlight recurring themes, and provide direct feedback on content. By analyzing these comments, brands can tailor their strategies to better meet audience expectations and foster deeper engagement.
Overview of TikTok's API Capabilities
TikTok's API offers a robust suite of tools for accessing various data points, including user profiles, video metadata, comments and hashtags from the TikTok web. These capabilities can significantly enhance TikTok scraping efforts, providing comprehensive inputs on user interactions and allowing you to extract data efficiently from posts. When making API requests, it's essential to define the right variables to ensure you retrieve the specific data you need.
Navigating TikTok's API
The TikTok API allows developers to access a wide array of information and scrape data, from basic user information to detailed video analytics. Understanding how to effectively navigate and utilize these endpoints is the first step toward successful data scraping.
Also read: How to use Tiktok API to Get Followers
What is a TikTok Comment Scraper?
A TikTok comment scraper is a tool designed to scrape TikTok data, specifically extracting comments from TikTok videos using an API. To avoid IP bans and ensure a smooth data extraction process, employing residential proxies can be highly effective.
This scraper automates the collection of comments from posts, saving time and effort while providing accurate and timely data for analysis. This data can be used for various purposes, such as enhancing engagement strategies, conducting market research, and improving content planning. With a well-designed scraper, you can automate the tedious process of data collection, allowing you to focus on analyzing the insights and making data-driven decisions.
Key Features of a Comment Scraper
- Automated Data Collection: Streamlines the process of gathering comments from published posts.
- Real-time Access: Provides up-to-date comments for immediate analysis.
- Data Storage: Organizes collected comments for easy retrieval and analysis.
- Scalability: Handles large volumes of data from multiple videos.
- Customization: Tailors data extraction based on specific needs.
Why Scraping TikTok Comments is Valuable
Enhancing Engagement and Feedback Analysis
Scraping data to analyze comments allows brands to gauge user sentiment and engagement levels, enabling more targeted and effective communication strategies. By understanding what TikTok users are saying about your content, you can make informed decisions to improve your overall engagement strategy.
Market Research and Trend Analysis
Scraping TikTok data helps identify emerging trends and preferences, informing product development and marketing campaigns. Staying ahead of trends is crucial in the fast-paced world of social media, and comments provide a direct line to what your audience cares about.
Content Strategy Improvement
Comment analysis reveals what content resonates with your audience, guiding future content creation and strategy. By understanding your audience's preferences, you can create content that not only engages but also drives meaningful interactions.
Free resource: Common Challenges with TikTok API and How to Overcome Them
How to Get Started with TikTok Comment Scraper API
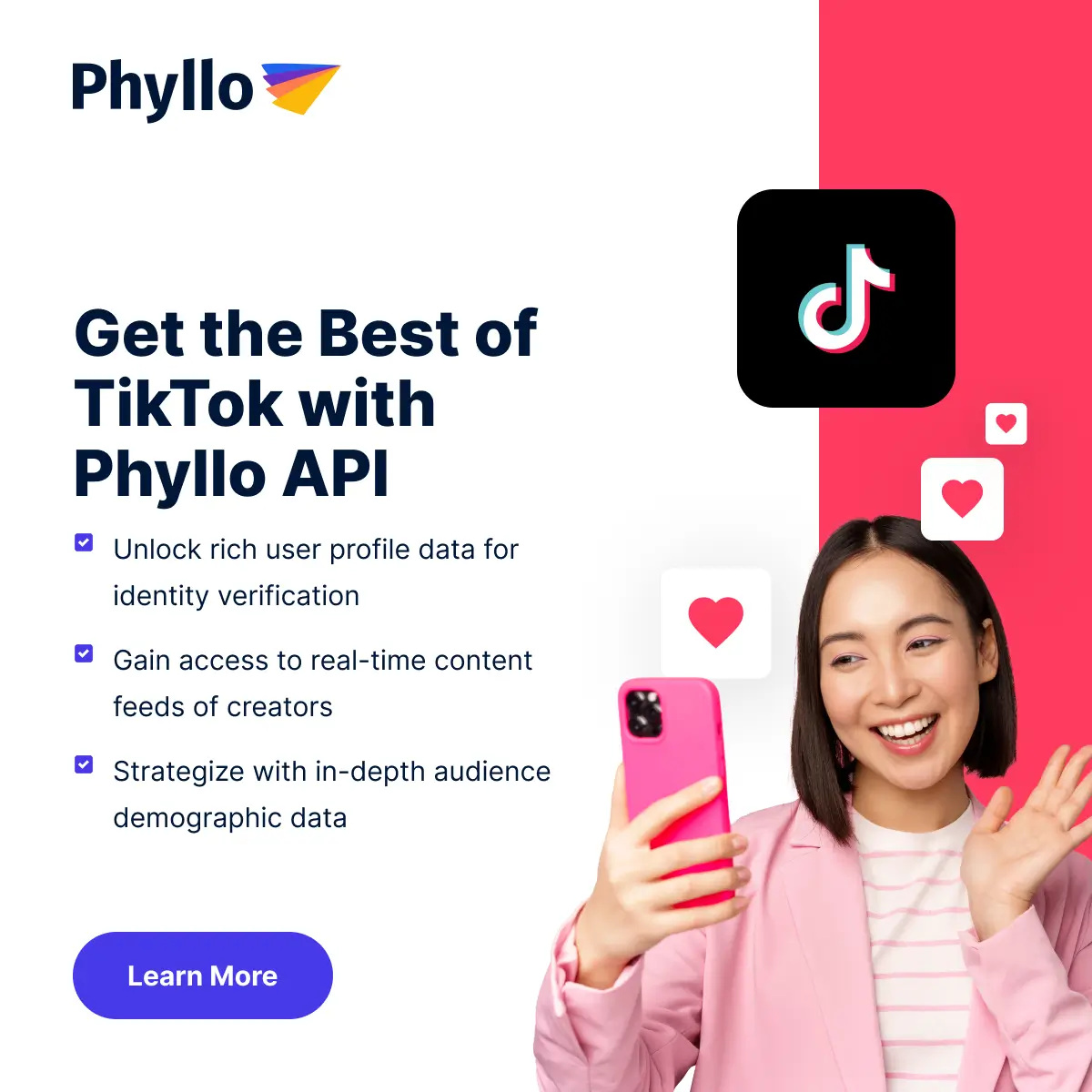
Setting Up Your Developer Account
To use TikTok's API, you need to set up a developer account. Visit TikTok's developer portal and follow the instructions to register your application. This process involves creating an account, registering your app, and obtaining the necessary API keys.
Setting up a developer account is straightforward. Start by visiting the TikTok Developer Portal, where you can sign up and create a new application. Once your application is registered, you will receive an API key and access token, which are essential for making API requests.
Obtaining API Access and Keys
After setting up your account, obtain the necessary API keys and access tokens. These credentials are essential for making authorized requests to TikTok's API. Ensure you store these credentials securely, as they are required for all API interactions.
To obtain API access, navigate to the "API Management" section in your developer account. Here, you can generate API keys and access tokens. Ensure you store these credentials securely, as they are required for all API interactions.
Also read: TikTok Influencer Analytics: How can brands find the right influencers?
Step-by-Step Guide to Using TikTok Comment Scraper API
To effectively perform TikTok scraping and extract all the data from TikTok Profiles, start by making basic API requests to fetch comments from specific videos. Analyze the API response to ensure that you are receiving the correct data. Utilizing residential proxies can help manage large volumes of requests without being blocked. Using programming languages like Python and other available resources can streamline the process. You can then save the extracted comments in different formats such as JSON or txt files for further analysis.
Basic API Requests for Comments
Start by making basic API requests to fetch comments and user information from TikTok profiles or from specific videos using a programming language like Python. Use endpoints provided by TikTok's API documentation to structure your requests. Here’s an example of how to make an API request to fetch comments:
import requests
# Replace 'your_api_key' with your actual API key
api_key = 'your_api_key'
video_id = 'example_video_id'
url = f'https://api.tiktok.com/comments?video_id={video_id}&api_key={api_key}'
response = requests.get(url)
comments = response.json()
for comment in comments['data']:
print(comment['text'])
This script makes a basic request to the TikTok API, retrieving comments for a specific video. The comments are then printed out, providing a simple way to access comment data.
Also read: Developer Guide on TikTok API Error Handling
Parsing and Storing Retrieved Data
Once comments are retrieved, parse the data to extract data and relevant information such as user IDs, comment text, and timestamps. Store this data in a structured format for easy access and analysis.
Use the code to parse and store the retrieved data:
import json
# Sample response data
response_data = '''{
"data": [
{"user_id": "12345", "text": "Great video!", "timestamp": "2023-07-23T12:34:56Z"},
{"user_id": "67890", "text": "Loved it!", "timestamp": "2023-07-23T12:35:56Z"}
]
}'''
# Parse JSON response
comments = json.loads(response_data)
# Store data in a structured format
comment_list = []
for comment in comments['data']:
comment_info = {
'user_id': comment['user_id'],
'text': comment['text'],
'timestamp': comment['timestamp']
}
comment_list.append(comment_info)
# Print stored comments
for comment in comment_list:
print(comment)
This example demonstrates how to parse a JSON response and store the extracted data in a structured format, such as a list of dictionaries.
Handling Rate Limits and Errors
TikTok's API imposes rate limits to prevent abuse. Implement error handling mechanisms to manage these limits and ensure uninterrupted data collection.
To handle rate limits, you can use a retry mechanism that waits before making additional requests using the code:
import time
def fetch_comments_with_retries(api_url, retries=3, wait=10):
for attempt in range(retries):
response = requests.get(api_url)
if response.status_code == 200:
return response.json()
elif response.status_code == 429:
print("Rate limit exceeded, waiting...")
time.sleep(wait)
else:
print(f"Error: {response.status_code}")
return None
comments = fetch_comments_with_retries(url)
This script retries the API request up to three times, waiting 10 seconds between attempts if a rate limit error occurs.
Integrating TikTok Comment Scraper with Data Analysis Tools
Once you've successfully extracted data from TikTok using a comment scraper, the next step is to analyze this data to gain actionable insights. The integration of your TikTok comment scraper with data analysis tools can enhance your ability to interpret the data and make informed decisions. This TikTok integration ensures seamless communication between your scraper and analytical tools, streamlining the process of transforming raw data into strategic insights.
Benefits of Data Analysis Integration
Integrating TikTok data with powerful data analysis tools like Excel, Google Sheets, or more advanced platforms like Tableau and Power BI allows you to visualize and interpret the data more effectively. This integration helps in identifying patterns, trends, and insights that may not be immediately apparent from raw data. Visualization tools can turn complex data sets into intuitive charts and graphs, making it easier to communicate findings and strategies to stakeholders.
Steps to Integrate TikTok Data with Analysis Tools
- Exporting Data:
- After extracting comments from TikTok, you can export the data into formats compatible with data analysis tools, such as CSV or Excel files. Python libraries like pandas can be used to format and export the data easily.
import pandas as pd
# Assuming comment_list is your list of comments with user_id, text, and timestamp
df = pd.DataFrame(comment_list)
df.to_csv('tiktok_comments.csv', index=False)
- Importing Data:
- Import the exported CSV file into your chosen data analysis tool. Most tools have straightforward import functions that allow you to upload your CSV file and start analyzing the data immediately.
- Data Cleaning and Preparation:
- Before analysis, clean the data to remove duplicates, handle missing values, and format the data correctly. This step ensures that your analysis is based on accurate and relevant data.
- Data Analysis and Visualization:
- Use the features of your data analysis tool to create visualizations such as bar charts, pie charts, and trend lines. For instance, you can visualize the frequency of certain keywords in comments, track engagement trends over time, or identify the most discussed topics.
import matplotlib.pyplot as plt
# Example of plotting the number of comments over time
df['timestamp'] = pd.to_datetime(df['timestamp'])
df.set_index('timestamp', inplace=True)
df['comment_count'] = 1
daily_comments = df['comment_count'].resample('D').sum()
plt.figure(figsize=(10, 5))
daily_comments.plot()
plt.title('Daily Number of Comments')
plt.xlabel('Date')
plt.ylabel('Number of Comments')
plt.show()
Advanced Analysis with Machine Learning
For more sophisticated analysis, consider integrating machine learning models to perform sentiment analysis, topic modeling, or predictive analytics. Python libraries such as scikit-learn and NLTK can be employed to build models that analyze the sentiment of comments or predict future engagement trends based on historical data.
Integrating TikTok comment data with advanced analysis tools and techniques allows for a deeper understanding of user interactions and can significantly enhance your content strategy, marketing efforts, and overall engagement on the platform. By leveraging these insights, you can tailor your content to better meet the needs and interests of your audience, ultimately driving greater success on TikTok.
Best Practices for Using TikTok Comment Scraper
Ensuring Data Privacy and Compliance
Adhere to data protection regulations and TikTok's terms of service to ensure compliance and avoid legal issues. Data privacy is a critical concern when scraping user comments. Always anonymize user data where possible and ensure your data collection practices comply with regulations such as GDPR and CCPA. Additionally, familiarize yourself with TikTok’s terms of service to avoid violating their policies.
When using a TikTok scraper, it's essential to consider data protection regulations such as GDPR (General Data Protection Regulation) and CCPA (California Consumer Privacy Act). These laws govern how personal data is collected, stored, and used. Always anonymize user data where possible and ensure your practices are compliant with these regulations.
Optimizing API Calls for Efficiency
Optimize your API calls to minimize latency and maximize data retrieval efficiency. Use batch requests where possible to reduce the number of API calls. Batch processing allows you to retrieve multiple sets of data in a single API call, reducing the load on TikTok’s servers and improving the efficiency of your scraper. Ensure your requests are as efficient as possible by only requesting the data you need for TikTok scraping and minimizing redundant calls. In our final thoughts, it's important to emphasize the value of adhering to these best practices for long-term success.
Regularly Updating Scraper for API Changes
Stay updated with TikTok's API changes to ensure your scraper continues to function correctly. Regular updates prevent disruptions in data collection. APIs are frequently updated to enhance functionality and security. Regularly check TikTok’s API documentation for updates and modify your scraper accordingly. This proactive approach ensures your data collection process remains smooth and uninterrupted.
How Phyllo API Enhances TikTok Comment Scraping
.webp)
Phyllo API streamlines the aggregation of comment data, making it easier to collect and conduct the scraping process from multiple videos.
Simplifying Data Aggregation with Phyllo
Phyllo’s robust data aggregation capabilities allow you to seamlessly gather comment data from various TikTok videos, providing a comprehensive view of user interactions. This simplifies the data collection process and enhances your ability to analyze trends and sentiment.
Improving Data Accuracy and Consistency
Phyllo ensures that the data collected is accurate and consistent, providing reliable insights for your analysis. Accurate and consistent data is crucial for making informed decisions. Phyllo’s API ensures that the data you collect is reliable, allowing you to trust your analysis and make data-driven decisions.
Enabling Easier Access and Enhanced Analysis
With Phyllo, accessing and analyzing comment data becomes straightforward, allowing for more in-depth and actionable insights. Phyllo’s API simplifies the process of accessing and analyzing comment data, making it easier to derive actionable insights. Whether you’re analyzing engagement trends or monitoring user sentiment, Phyllo provides the tools you need to enhance your analysis.
Use Cases: Enhanced Analytics and Reporting with Phyllo Integration
Integrating Phyllo API with your data scraping efforts can significantly enhance your analytics and reporting capabilities, providing a comprehensive view of user interactions. Phyllo’s integration allows for enhanced analytics and reporting, providing a comprehensive view of user interactions. This holistic view enables you to make more informed decisions and optimize your strategies.
Conclusion
Scraping TikTok comments using an API provides valuable insights into user engagement and preferences. By following best practices and leveraging tools like Phyllo API, along with Python and other valuable resources, you can optimize your data collection and analysis processes for an efficient and effective scraping process. Start implementing TikTok comment scraping today to enhance your content strategy and market research efforts.
Phyllo has managed to build deep coverage across all major platforms used by your customers.
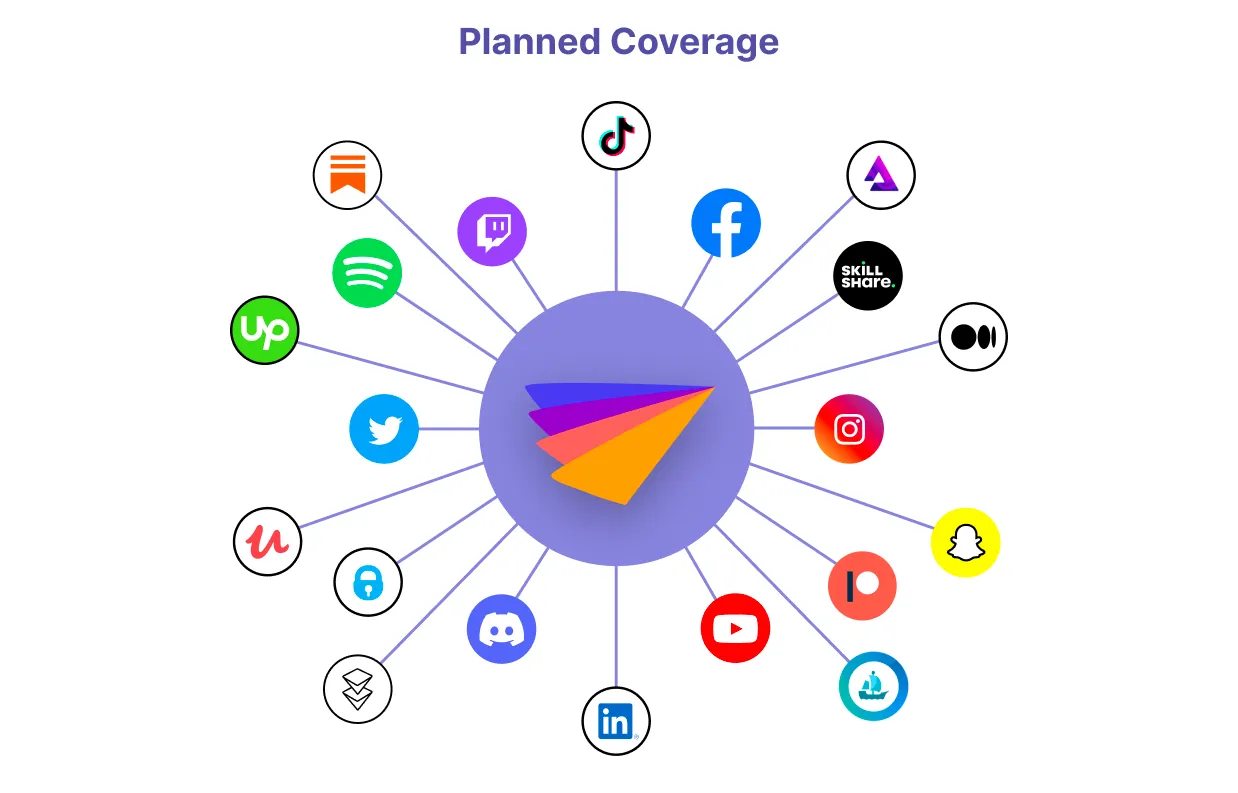
Check it out here.
