Hey there! So, you’re curious about how to use the Twitch API to get your follower count? Maybe you're a developer who wants to integrate Twitch data into your app, or you just want to keep an eye on your follower stats. Whatever the reason, you’ve come to the right place. In this guide, we’re going to walk you through everything you need to know about tracking your channel followers and accessing the necessary URLs..
Twitch API: What It Is
First things first, what exactly is the Twitch API? Simply put, it’s a tool that lets you access all sorts of Twitch data programmatically. This can be super handy for tracking metrics like follower count, which is a key indicator of how popular your Twitch channel is.
Think of the Twitch API as a bridge that connects your application to Twitch’s vast sea of data. It has various endpoints, each serving up different slices of data. Whether you’re looking for user info, live stream details, video content, or channel followers metrics by username, the Twitch API has got you covered, including data related to the game being streamed.
Why Your Follower Count Matters
Why should you care about your follower count? Well, it’s a great way to measure your channel’s growth and popularity. Plus, if you’re a developer, having access to real-time follower data can help you build some pretty cool tools for streamers.
Understanding OAuth and Authentication
Before we get our hands dirty with the API, there’s something important we need to talk about: OAuth. This is the authentication protocol that Twitch uses. In simple terms, OAuth lets applications access user data without needing to know their passwords. When you use the Twitch API, you’ll need to authenticate your app and get an OAuth token. This token is like a key that unlocks access to specific parts of a user’s account.
How to Use Twitch API to Get Followers
Step 1: Create a New Application on the Twitch Developer Dashboard
Ready to get started? The first thing you need to do is create a new application on the Twitch Developer Dashboard. Here’s how:
- Log in to your Twitch account and head over to the Developer Dashboard.
- Click on the “Register Your Application” button.
- Fill in the necessary fields like the application's name, OAuth redirect URL, and category.
- Save your changes to get your client ID.
Tips for Creating Your Application
Creating an app on the Twitch Developer Dashboard is pretty straightforward, but here are a few tips to keep in mind. Make sure your app’s name is descriptive enough so you remember its purpose later on. The OAuth redirect URL is super important; this is where users will be sent after they authorize your app. Double-check this URL to make sure it’s correct and secure.
Step 2: Get Your OAuth Token
Next up, you’ll need an OAuth token to access the Twitch API. Here’s what you do:
- Go to the Twitch Token Generator and log in with your Twitch account.
- Authorize the application to access your account.
- Copy the OAuth token provided.
Generating Your OAuth Token
Getting your OAuth token might sound a bit technical, but it’s actually quite simple. The token generator will guide you through the process. Remember, this token is your golden ticket to accessing Twitch data, so keep it safe and don’t share it with anyone.
Step 3: Make a Request to the Twitch API
Now that you have your client ID and OAuth token, it’s time to make a request to the Twitch API to get the follower count. Here’s a quick example using Python:
import requests
client_id = 'your_client_id'
oauth_token = 'your_oauth_token'
headers = {
'Client-ID': client_id,
'Authorization': f'Bearer {oauth_token}'
}
url = 'https://api.twitch.tv/helix/users/follows?to_id=your_channel_id'
response = requests.get(url, headers=headers)
data = response.json()
follower_count = data['total']
print(f'Follower count: {follower_count}')
Breaking Down the Code
Let’s break down what’s happening in this script:
- Import Requests Library: This handy library helps you send HTTP requests in Python.
- Set Up Authentication Headers: You need to include your client ID and OAuth token in the headers to authenticate your request.
- Construct the API URL: This URL points to the endpoint for retrieving follower data.
- Send the Request: The request is sent to the Twitch API, and the response is captured.
- Parse the Response: The JSON response is parsed to extract the follower count.
Step 4: Handle API Rate Limits
A quick heads-up: Twitch has API rate limits, which means you can only make a certain number of requests in a given time period. If you go over this limit, you’ll get errors. Here’s how to manage it:
Understanding Rate Limits and Best Practices
Rate limits ensure everyone gets fair access to the API. Each API key has a cap on how many requests it can make in a specific time frame. If you exceed this, you’ll hit an HTTP 429 error. Here’s what you can do:
- Monitor Your Requests: Keep track of your request count to stay within the limits.
- Implement Exponential Backoff: If you hit the limit, wait a bit before retrying, increasing the wait time each attempt.
- Optimize Your Requests: Combine multiple data needs into single requests where possible.
Step 5: Troubleshooting Common Issues
You might run into some common issues. Don’t worry; here’s how to tackle them:
- Error: Unauthorized (401): Make sure your OAuth token is valid and has the necessary permissions.
- Error: Too Many Requests (429): You’ve hit the rate limit. Use a retry strategy with exponential backoff.
- Error: Not Found (404): Check if the channel ID is correct.
Detailed Troubleshooting Tips
- Unauthorized Error (401): This means your OAuth token might be expired or missing the required permissions. Re-authenticate and double-check your token.
- Too Many Requests (429): Slow down your requests. Implement a retry mechanism with increasing delays.
- Not Found (404): Make sure the channel ID is correct. It should be a numerical ID corresponding to a valid Twitch channel.
Example Scripts
Here are some example scripts to help you get started:
Python
import requests
def get_follower_count(client_id, oauth_token, channel_id):
headers = {
'Client-ID': client_id,
'Authorization': f'Bearer {oauth_token}'
}
url = f'https://api.twitch.tv/helix/users/follows?to_id={channel_id}'
response = requests.get(url, headers=headers)
data = response.json()
return data['total']
client_id = 'your_client_id'
oauth_token = 'your_oauth_token'
channel_id = 'your_channel_id'
follower_count = get_follower_count(client_id, oauth_token, channel_id)
print(f'Follower count: {follower_count}')
Integrating with Your Application
![How To Mod Someone On Twitch [2024 Guide] - PC Strike](https://cdn.prod.website-files.com/6284f7327b63a63510a796c8/66c4bbae715cfb9046a23a6e_How-To-Mod-Someone-On-Twitch-600x338.png)
Once you have the follower count, you can integrate this data into your application. Whether you’re building a dashboard, a chat bot, or any other tool, having access to real-time follower counts can enhance your application’s functionality and user experience.
Practical Applications and Use Cases
- Streamer Dashboards: Create a real-time dashboard for streamers to monitor their follower growth.
- Chat Bots: Develop chat bots that announce new follower milestones or provide follower counts on demand.
- Analytics Tools: Integrate follower data into analytics tools to track growth trends and engagement metrics.
Best Practices for Using the Twitch API
To make the most of the Twitch API, consider these best practices:
Secure Your Credentials: Always keep your client ID and OAuth token secure. Never expose them in client-side code.
Respect Rate Limits: Adhere to the rate limits to avoid disruptions in service and potential bans.
Handle Errors Gracefully: Implement robust error handling to manage API errors and provide a smooth user experience.
Stay Updated: Regularly check the Twitch API documentation for updates and new features.
Conclusion
Using the Twitch API to retrieve follower counts is a straightforward process that requires setting up an application, obtaining an OAuth token, and making a request to the appropriate endpoint. By following this guide, you should be able to integrate Twitch follower data into your application effectively. Remember to handle API rate limits and common errors to ensure a smooth and reliable experience.
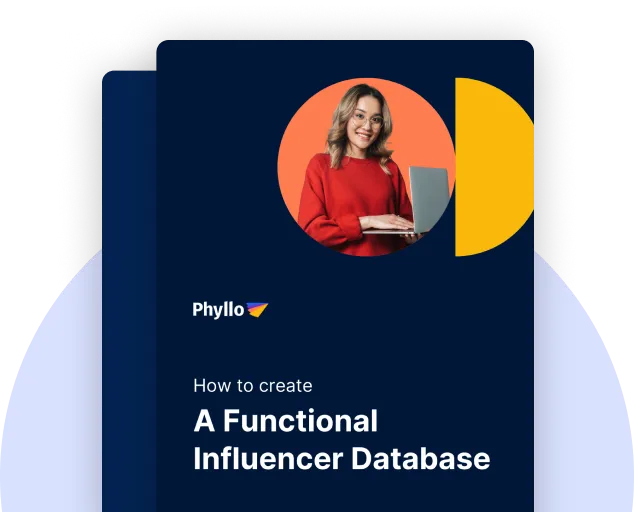